forked from IanF/OneWireArduinoSlaveATTINY85
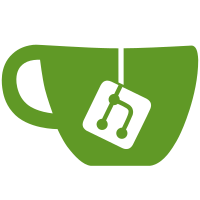
11 changed files with 287 additions and 32 deletions
@ -1,29 +1,72 @@
|
||||
#include "Arduino.h" |
||||
#include "SerialChannel.h" |
||||
|
||||
short SerialChannel::nextId = 0; |
||||
byte SerialChannel::nextId = 1; |
||||
SerialChannel* SerialChannel::first = 0; |
||||
|
||||
SerialChannel::SerialChannel() |
||||
SerialChannel::SerialChannel(const char* name_) |
||||
: next(0) |
||||
, id((byte)-1) |
||||
, name(name_) |
||||
{ |
||||
if(first == 0) |
||||
first = this; |
||||
else |
||||
{ |
||||
SerialChannel* c = first; |
||||
while(c->next != 0) c = c->next; |
||||
c->next = this; |
||||
} |
||||
|
||||
id = nextId++; |
||||
} |
||||
|
||||
void SerialChannel::init(const char* name) |
||||
void SerialChannel::write(byte* data, short byteCount, unsigned long time) |
||||
{ |
||||
id = nextId++; |
||||
Serial.write((short)0); |
||||
if (time == (unsigned long)-1) |
||||
time = micros(); |
||||
|
||||
handleConnection(); |
||||
|
||||
Serial.write("START"); |
||||
Serial.write(id); |
||||
Serial.write(strlen(name)); |
||||
Serial.write(name); |
||||
writeULong(time); |
||||
writeShort(byteCount); |
||||
Serial.write(data, byteCount); |
||||
} |
||||
|
||||
void SerialChannel::write(byte* data, short byteCount) |
||||
void SerialChannel::write(const char* text, unsigned long time) |
||||
{ |
||||
Serial.write(byteCount); |
||||
Serial.write(id); |
||||
Serial.write(data, byteCount); |
||||
write((byte*)text, strlen(text), time); |
||||
} |
||||
|
||||
void SerialChannel::write(const char* text) |
||||
void SerialChannel::writeShort(short num) |
||||
{ |
||||
Serial.write((byte*)text, strlen(text)); |
||||
Serial.write((byte*)&num, 2); |
||||
} |
||||
|
||||
void SerialChannel::writeULong(unsigned long num) |
||||
{ |
||||
Serial.write((byte*)&num, 4); |
||||
} |
||||
|
||||
void SerialChannel::handleConnection() |
||||
{ |
||||
int b = Serial.read(); |
||||
if(b == (int)'C') |
||||
{ |
||||
Serial.write("CONNECTION"); |
||||
SerialChannel* c = first; |
||||
while(c) |
||||
{ |
||||
Serial.write("START"); |
||||
Serial.write(0); |
||||
Serial.write("ChannelInit"); |
||||
Serial.write(c->id); |
||||
writeShort(strlen(c->name)); |
||||
Serial.write(c->name); |
||||
c = c->next; |
||||
} |
||||
} |
||||
} |
||||
|
||||
|
@ -1,8 +1,11 @@
|
||||
<Window x:Class="SerialMonitor.MainWindow" |
||||
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" |
||||
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" |
||||
Title="MainWindow" Height="350" Width="525"> |
||||
Title="Serial Monitor" Height="396" Width="629"> |
||||
<Grid> |
||||
|
||||
<TextBlock TextWrapping="Wrap" VerticalAlignment="Bottom" Height="150" Text="{Binding ConsoleText}" Style="{DynamicResource Console}" Grid.ColumnSpan="2"/> |
||||
<ScrollViewer HorizontalScrollBarVisibility="Visible" Margin="0,0,0,155" Grid.ColumnSpan="2"> |
||||
<Canvas Style="{DynamicResource Oscilloscope}"/> |
||||
</ScrollViewer> |
||||
</Grid> |
||||
</Window> |
||||
|
@ -0,0 +1,40 @@
|
||||
using System; |
||||
using System.Collections.Generic; |
||||
using System.ComponentModel; |
||||
using System.Linq; |
||||
using System.Text; |
||||
using System.Threading.Tasks; |
||||
|
||||
namespace SerialMonitor |
||||
{ |
||||
public class MainWindowContext : INotifyPropertyChanged |
||||
{ |
||||
public MainWindowContext() |
||||
{ |
||||
Get = this; |
||||
} |
||||
|
||||
public static MainWindowContext Get { get; private set; } |
||||
|
||||
private List<string> Lines = new List<string>(); |
||||
public string ConsoleText { get { return String.Join("\n", Lines); } } |
||||
|
||||
public void WriteLine(string line) |
||||
{ |
||||
Lines.Add(line); |
||||
if (Lines.Count > 9) |
||||
Lines.RemoveAt(0); |
||||
OnPropertyChanged("ConsoleText"); |
||||
} |
||||
|
||||
public event PropertyChangedEventHandler PropertyChanged; |
||||
protected void OnPropertyChanged(string name) |
||||
{ |
||||
PropertyChangedEventHandler handler = PropertyChanged; |
||||
if (handler != null) |
||||
{ |
||||
handler(this, new PropertyChangedEventArgs(name)); |
||||
} |
||||
} |
||||
} |
||||
} |
@ -0,0 +1,26 @@
|
||||
using System; |
||||
using System.Collections.Generic; |
||||
using System.Linq; |
||||
using System.Text; |
||||
using System.Threading.Tasks; |
||||
|
||||
namespace SerialMonitor |
||||
{ |
||||
public class SerialMessage |
||||
{ |
||||
public string ChannelName { get; set; } |
||||
|
||||
/// <summary> |
||||
/// Time at which the message was sent, in microseconds since the start of the remote executable |
||||
/// </summary> |
||||
public ulong SendTime { get; set; } |
||||
|
||||
public byte[] Data { get; set; } |
||||
|
||||
public string StringData |
||||
{ |
||||
get { return Encoding.UTF8.GetString(Data); } |
||||
set { Data = Encoding.UTF8.GetBytes(value); } |
||||
} |
||||
} |
||||
} |
@ -0,0 +1,44 @@
|
||||
using System; |
||||
using System.Collections.Generic; |
||||
using System.IO.Ports; |
||||
using System.Linq; |
||||
using System.Text; |
||||
using System.Threading.Tasks; |
||||
|
||||
namespace SerialMonitor |
||||
{ |
||||
public static class SerialPortExtensions |
||||
{ |
||||
public static int ReadShort(this SerialPort port) |
||||
{ |
||||
int b1 = port.ReadByte(); |
||||
int b2 = port.ReadByte(); |
||||
return b2 * 256 + b1; |
||||
} |
||||
|
||||
public static ulong ReadULong(this SerialPort port) |
||||
{ |
||||
ulong b1 = (ulong)port.ReadByte(); |
||||
ulong b2 = (ulong)port.ReadByte(); |
||||
ulong b3 = (ulong)port.ReadByte(); |
||||
ulong b4 = (ulong)port.ReadByte(); |
||||
return b4 * 256 * 256 * 256 + b3 * 256 * 256 + b2 * 256 + b1; |
||||
} |
||||
|
||||
public static byte[] ReadBytes(this SerialPort port, int numBytes) |
||||
{ |
||||
var bytes = new byte[numBytes]; |
||||
int remaining = numBytes; |
||||
int pos = 0; |
||||
while (remaining > 0) |
||||
{ |
||||
int read = port.Read(bytes, pos, remaining); |
||||
if (read < 0) |
||||
throw new Exception("Connection closed"); |
||||
remaining -= read; |
||||
pos += read; |
||||
} |
||||
return bytes; |
||||
} |
||||
} |
||||
} |
Loading…
Reference in new issue